BASICS OF JavaScript PROGRAMMING LANGUAGE
JavaScript IMPLEMENTATIONS
A Complete JavaScript implementations is made up of three distinct parts:
- The core (ECMAScript)
- The document Object Model (DOM)
- The Browser Object MOdel (BOM)
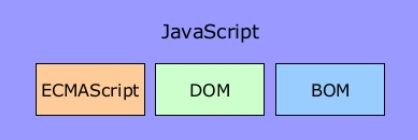
JavaScript DEFINITION OF ECMA Script
ECMAScript is simply a description, defining all the properties, methods and objects of a scripting language.
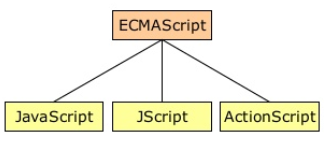
- Syntax
- Types
- Statements
- Keywords
- Reserved words
- Operators
- Objects
Each browser has it own implementation of the ECMAScript interface, which is then extended of contain the DOM and BOM. Today, all popular Web browsers comply with the 3rd edition of ECMA-262.
JavaScript DEFINITION OF DOCUMENT OBJECT MODEL (DOM)
The Document Object Model (DOM) describes methods and interfaces for working the content of a Web page.
- The DOM is an three-based, language independent API for HTML as well as XML. (cf. The SAX provides an event-based API to parse XML.)
- The W3C DOM specifications: Level1, Level2, Level3
- The document object is the only object that belongs to both the DOM and the BOM
getElementsByTagName(), getElementsByName(),getElementById()
All attributes are included in HTML DOM elements as properties
oImg.src = "mypicture.jpg";
oDiv.className = "footre";
JavaScript DEFINITION OF BROWSER OBJECT MODEL (BOM)
The Browser Object Model (BOM) describes methods and interfaces for interacting with the browser.
Because no standards exist for the BOM, each browser has it own implementations.
The window object represents an entire browser window.
objects: document (anchors, forms, images, links, location);
frames (history, location, navigator, screen).
methods: moveBy(), moveTo(), resizeBy(), resizeTo(), open(), close(), alert(), confirm(), input(), setTimeOut(), clearTimeOut(), setInterval(), clearInterval()
properties: screenX, screenY, status, defaultStatus, etc.
JavaScript BASICS (ECMaScript)
JavaScript SYNTAX
JavaScript borrows most of its syntax from Java, but also inherits from Awk and Perl, with some inherits influence from Self in its object protype system.
JavaScript THE BASIC CONCEPTS
Everything is case-sensitive
Variables are loosely typed.
- Use the var keyword
- Variables don't have to be declared before being used.
End-of-line semicolons are optional
var test1 = "red"
var test2 = "blue"; // do this to avoid confusion"
- Comments are the same as in Java, C and Perl.
- Braces indicade code blocks
JavaScript KEYWORDS & RESERVED WORDS
The keywords and reserved words cannot be used as variables or function names.
Keywords: break, case, catch, continue, default, delete, do, else, finally, for, function, if, in, instanceof, new, return, switch, this, throw, try, typeof, var, void, while, with.
Reserved words: abstract, boolean, byte, char, class, const, debugger, double, enum, export, extends, final, float, goto, implements, import, int, interface, long, native, package, private, protected, public, short, static, super, synchronized, throws, transient, volatile.
JavaScript PRIMITIVE AND REFERENCE VALUES
JavaScript PRIMITIVE VALUES
Primitive Values are simple pieces of data that are stored on the stack.
The value is one of the JavaScript primitive types: Undefined, Null, Boolean, Number, or String.
Many languages consider strings as a reference type and not a primitive type, but JavaScript breaks from this tradition.
JavaScript PRIMITIVE TYPES
JavaScript has five primitive types:
Undefined - The undefined type has only one value, undefined.
Null - The Null type has only one value, null.
Boolean - The Boolean type has two values, true and false.
Number - 32-bit integer and 64-bit floating-point values.
String - Using either double quotes("") or single quote(')
JavaScript REFERENCE VALUES
Reference values are objects that are stored in the heap, meaning that the value stored in the variable location is a pointer to a location in memory where the object is stored.
JavaScript THE typeof OPERATOR
JavaScript to determine if a value is in the range of values for a particular type, provies the typeof operator.
value | typeof |
---|---|
Boolean | boolean |
Number | number |
String | string |
Undefined | undefined |
Null | object |
Object | object |
Array | object |
Function | function |
JavaScript CONVERSIONS
JavaScript CONVERTING TO A STRING
Primitive values for booleans, number, strings are pseudo-objects, which means they actually have properties and methods.
JavaScript CONVERTING TO A NUMBER
JavaScript provides two methods for converting non-number primitives into numbers: parseInt() and parseFloat().var numb1 = parseInt("0xA"); // returns 10 var num2 = parseFloat("4.5.6"); // returns 4.5 var num3 = parseInt("blue"); // returns NaN
JavaScript TYPE CASTING
JavaScript BOOLEAN (value)
Boolean("") → false; Boolean("hi") → trueBoolean(0) → false; Boolean(100) → true
Boolean(null) → false; Boolean(undefined) → false
Boolean(new Object()) → true
JavaScript NUMBER (value)
Number(false) → 0; Number(true) → 1Number(null) → 0; Number(undefined) → NaN
Number("4.5.6") → NaN (cf. parseFloat))
Number(new Object()) → NaN
JavaScript STRING (value)
String(null) → "null"String(undefined) → "undefined"
JavaScript REFERENCE TYPES
Reference types are comonly reffered to as classes, which is to say that when you have a reference value, you are dealing with an object.ECMAScript defines "object definitions" that are logically equivalent to "classes" in other programming languages.
The new operator
var obj = new Object:
var obj = new Object(); //do this to avoid confusion.
THE instanceof OPERATOR
The instanceof operator identifies the type of object you are working with.var aStringObject = new String ("Hello");
var result = (aStringObject instanceof String); //returns true
No comments:
Post a Comment